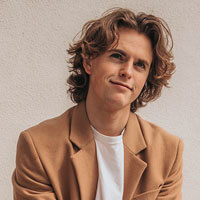
-
John DoePro
johndoe@gmail.com - Profile
- Inbox
- Lock Screen
- Sign Out
Simple Table
CodeName | Date | Sale | Status | Action |
---|---|---|---|---|
<!-- basic table --> <div class="table-responsive"> <table> <thead> <tr> <th>Name</th> <th>Date</th> <th>Sale</th> <th class="text-center">Status</th> <th class="text-center">Action </th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td x-text="data.name" class="whitespace-nowrap"></td> <td x-text="data.date"></td> <td x-text="data.sale"></td> <td class="text-center whitespace-nowrap" :class="{'text-success': data.status === 'Complete', 'text-secondary': data.status === 'Pending', 'text-info': data.status === 'In Progress', 'text-danger': data.status === 'Canceled'}" x-text="data.status"></td> <td class="text-center"> <button type="button" x-tooltip="Delete"> <svg> ... </svg> </button> </td> </tr> </template> </tbody> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
Hover Table
CodeName | Date | Sale | Status | Action |
---|---|---|---|---|
<!-- hover table --> <div class="table-responsive"> <table class="table-hover"> <thead> <tr> <th>Name</th> <th>Date</th> <th>Sale</th> <th class="text-center">Status</th> <th class="text-center">Action</th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td x-text="data.name" class="whitespace-nowrap"></td> <td x-text="data.date"></td> <td x-text="data.sale"></td> <td class="text-center whitespace-nowrap" :class="{'text-success': data.status === 'Complete', 'text-secondary': data.status === 'Pending', 'text-info': data.status === 'In Progress', 'text-danger': data.status === 'Canceled'}" x-text="data.status"></td> <td class="text-center"> <button type="button" x-tooltip="Delete"> <svg> ... </svg> </button> </td> </tr> </template> </tbody> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
Striped Table
CodeName | Date | Sale | |
---|---|---|---|
<!-- striped table --> <div class="table-responsive"> <table class="table-striped"> <thead> <tr> <th>Name</th> <th>Date</th> <th>Sale</th> <th></th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td x-text="data.name" class="whitespace-nowrap"></td> <td x-text="data.date"></td> <td x-text="data.sale"></td> <td class="text-center"> <button type="button" x-tooltip="Delete"> <svg> ... </svg> </button> </td> </tr> </template> </tbody> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
Table Light
Code# | Name | Created At | ||
---|---|---|---|---|
<!-- table light --> <div class="table-responsive"> <table class="table-hover"> <thead> <tr class="!bg-transparent dark:!bg-transparent"> <th>#</th> <th>Name</th> <th>Email</th> <th>Created At</th> <th class="text-center"></th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td x-text="data.id"></td> <td x-text="data.name" class="whitespace-nowrap"></td> <td x-text="data.email"></td> <td x-text="data.date"></td> <td class="text-center"> <button type="button" x-tooltip="Delete"> <svg> ... </svg> </button> </td> </tr> </template> </tbody> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
Captions
Code# | Name | Status | Register | |
---|---|---|---|---|
<!-- caption --> <div class="table-responsive"> <table> <thead> <tr> <th>#</th> <th>Name</th> <th>Email</th> <th>Status</th> <th class="text-center">Register</th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td x-text="data.id"></td> <td x-text="data.name" class="whitespace-nowrap"></td> <td x-text="data.email"></td> <td> <span class="badge whitespace-nowrap" :class="{'badge-outline-primary': data.status === 'Complete', 'badge-outline-secondary': data.status === 'Pending', 'badge-outline-info': data.status === 'In Progress', 'badge-outline-danger': data.status === 'Canceled'}" x-text="data.status"></span></td> <td class="text-center" x-text="data.register"></td> </tr> </template> </tbody> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
Progress Table
Code# | Name | Progress | Sales | Action |
---|---|---|---|---|
|
<!-- progress table --> <div class="table-responsive"> <table> <thead> <tr> <th>#</th> <th>Name</th> <th>Progress</th> <th>Sales</th> <th class="text-center">Action</th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td x-text="data.id"></td> <td x-text="data.name" class="whitespace-nowrap"></td> <td> <div class="h-1.5 bg-[#ebedf2] dark:bg-dark/40 rounded-full flex w-full"> <div class="h-1.5 rounded-full rounded-bl-full text-center text-white text-xs" :class="{'bg-success': data.status === 'Complete', 'bg-secondary': data.status === 'Pending', 'bg-info': data.status === 'In Progress', 'bg-danger': data.status === 'Canceled'}" :style="`width: ${data.progress}`"></div> </div> </td> <td x-text="data.progress" class="whitespace-nowrap" :class="{'text-success': data.status === 'Complete', 'text-secondary': data.status === 'Pending', 'text-info': data.status === 'In Progress', 'text-danger': data.status === 'Canceled'}"></td> <td class="p-3 border-b border-[#ebedf2] dark:border-[#191e3a] text-center"> <button type="button" x-tooltip="Edit"> <svg> ... </svg> </button> <button type="button" x-tooltip="Delete"> <svg> ... </svg> </button> </td> </tr> </template> </tbody> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
Contextual
Code# | First Name | Last Name | |
---|---|---|---|
1 | John | Doe | johndoe@yahoo.com |
2 | Andy | King | andyking@gmail.com |
3 | Lisa | Doe | lisadoe@yahoo.com |
4 | Vincent | Carpenter | vinnyc@yahoo.com |
5 | Amy | Diaz | amydiaz@yahoo.com |
6 | Nia | Hillyer | niahill@gmail.com |
7 | Marry | McDonald | marryMcD@yahoo.com |
8 | Shaun | Park | park@yahoo.com |
<!-- contextual --> <div class="table-responsive"> <table> <thead> <tr> <th>#</th> <th>First Name</th> <th>Last Name</th> <th>Email</th> </tr> </thead> <tbody> <tr class="bg-dark-dark-light border-dark-dark-light"> <td>1</td> <td>John</td> <td>Doe</td> <td>johndoe@yahoo.com</td> </tr> <tr class="bg-primary/20 border-primary/20"> <td>2</td> <td>Andy</td> <td>King</td> <td>andyking@gmail.com</td> </tr> <tr class="bg-secondary/20 border-secondary/20"> <td>3</td> <td>Lisa</td> <td>Doe</td> <td>lisadoe@yahoo.com</td> </tr> <tr class="bg-success/20 border-success/20"> <td>4</td> <td>Vincent</td> <td>Carpenter</td> <td>vinnyc@yahoo.com</td> </tr> <tr class="bg-dark-dark-light border-dark-dark-light"> <td>5</td> <td>Amy</td> <td>Diaz</td> <td>amydiaz@yahoo.com</td> </tr> <tr class="bg-danger/20 border-danger/20"> <td>6</td> <td>Nia</td> <td>Hillyer</td> <td>niahill@gmail.com</td> </tr> <tr class="bg-info/20 border-info/20"> <td>7</td> <td>Marry</td> <td>McDonald</td> <td>marryMcD@yahoo.com</td> </tr> <tr class="bg-warning/20 border-warning/20"> <td>8</td> <td>Shaun</td> <td>Park</td> <td>park@yahoo.com</td> </tr> </tbody> </table> </div>
Dropdown
Code<!-- dropdown --> <div class="table-responsive"> <table> <thead> <tr> <th>Name</th> <th>Date</th> <th>Sale</th> <th>Status</th> <th class="text-center">Action</th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td x-text="data.name" class="whitespace-nowrap"></td> <td x-text="data.date"></td> <td x-text="data.sale"></td> <td> <span class="badge whitespace-nowrap" :class="{'bg-primary': data.status === 'Complete', 'bg-secondary': data.status === 'Pending', 'bg-success': data.status === 'In Progress', 'bg-danger': data.status === 'Canceled'}" x-text="data.status"></span></td> <td class="text-center"> <div x-data="dropdown" @click.outside="open = false" class="dropdown"> <a href="javascript:;" class="inline-block" @click="toggle"> <svg> ... </svg> </a> <ul x-cloak x-show="open" x-transition x-transition.duration.300ms class="ltr:right-0 rtl:left-0"> <li><a href="javascript:;" @click="toggle">Download</a></li> <li><a href="javascript:;" @click="toggle">Share</a></li> <li><a href="javascript:;" @click="toggle">Edit</a></li> <li><a href="javascript:;" @click="toggle">Delete</a></li> </ul> </div> </td> </tr> </template> </tbody> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
Table with Footer
Code<!-- table with footer --> <div class="table-responsive"> <table> <thead> <tr> <th>Name</th> <th>Position</th> <th>Office</th> <th class="text-center">Action</th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td x-text="data.name" class="whitespace-nowrap"></td> <td x-text="data.position"></td> <td x-text="data.office"></td> <td class="text-center"> <ul class="flex items-center justify-center gap-2"> <li> <a href="javascript:;" x-tooltip="Edit"> <svg> ... </svg> </a> </li> <li> <a href="javascript:;" x-tooltip="Delete"> <svg> ... </svg> </a> </li> </ul> </td> </tr> </template> </tbody> <tfoot> <tr> <th> <div>Name</div> </th> <th> <div>Position</div> </th> <th> <div>Office</div> </th> <th class="p-3 text-center"> <div>Action</div> </th> </tr> </tfoot> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
Checkboxes
Code<!-- checkbox --> <div class="table-responsive"> <table> <thead> <tr> <th><input type="checkbox" class="form-checkbox" /></th> <th>Name</th> <th>Date</th> <th>Sale</th> <th class="!text-center">Action</th> </tr> </thead> <tbody> <template x-for="data in tableData" :key="data.id"> <tr> <td><input type="checkbox" class="form-checkbox" /></td> <td x-text="data.name" class="whitespace-nowrap"></td> <td x-text="data.date"></td> <td x-text="data.sale"></td> <td class="text-center"> <ul class="flex items-center justify-center gap-2"> <li> <a href="javascript:;" x-tooltip="Settings"> <svg> ... </svg> </a> </li> <li> <a href="javascript:;" x-tooltip="Edit"> <svg> ... </svg> </a> </li> <li> <a href="javascript:;" x-tooltip="Delete"> <svg> ... </svg> </a> </li> </ul> </td> </tr> </template> </tbody> </table> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ tableData: [{ id: 1, name: 'John Doe', email: 'johndoe@yahoo.com', date: '10/08/2020', sale: 120, status: 'Complete', register: '5 min ago', progress: '40%', position: 'Developer', office: 'London' }, ...... })); }); </script>
©
. Vristo All rights reserved.