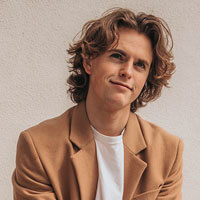
-
John DoePro
johndoe@gmail.com - Profile
- Inbox
- Lock Screen
- Sign Out
- Forms
- TouchSpin
Button Spin
Code<!-- basic --> <div class="flex" x-data="touchspin"> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-primary" @click="value1--;if(value1 < 0) value1 = 0;"> <svg> ... </svg> </button> <input type="number" placeholder="55" x-model="value1" class="form-input rounded-none text-center" min="0" max="100" readonly readonly @wheel="changeValue($event, 'value1')" /> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-primary" @click="value1++; if(value1 > 100) value1 = 100;"> <svg> ... </svg> </button> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("touchspin", () => ({ value1: 0, changeValue(e, val) { e.preventDefault(); if (e.deltaY < 0) { this.value1++; this.value1 > 100 ? this.value1 = 100 : this.value1; } else { this.value1--; this.value1 < 0 ? this.value1 = 0 : this.value1; } } })); }); </script>
Spin button with step of 5
Code<!-- step of 5 --> <div class="flex" x-data="touchspin"> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-primary" @click="value2 -=5 ;if(value2 < 0) value2 = 0;"> <svg> ... </svg> </button> <input type="number" x-model="value2" placeholder="5" step="5" min="0" max="50" readonly class="form-input rounded-none text-center" @wheel="changeValue($event, 'value2')" /> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-primary" @click="value2 += 5; if(value2 > 50) value2 = 50;"> <svg> ... </svg> </button> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("touchspin", () => ({ value2: 0, changeValue(e, val) { e.preventDefault(); if (e.deltaY < 0) { this.value2 += 5; this.value2 > 50 ? this.value2 = 50 : this.value2; } else { this.value2 -= 5; this.value2 < 0 ? this.value2 = 0 : this.value2; } } })); }); </script>
Wrapping value spin button
Code<!-- wrapping value --> <div class="flex" x-data="touchspin"> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-primary" @click="value3 -=1 ;if(value3 < 0) value3 = 20;"> <svg> ... </svg> </button> <input type="number" x-model="value3" placeholder="_ _" class="form-input rounded-none text-center" min="0" max="20" readonly @wheel="changeValue($event, 'value3')" /> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-primary" @click="value3 += 1; if(value3 > 20) value3 = 0;"> <svg> ... </svg> </button> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("touchspin", () => ({ value3: 0, changeValue(e, val) { e.preventDefault(); if (e.deltaY < 0) { this.value3 += 1; this.value3 > 20 ? this.value3 = 0 : this.value3; } else { this.value3 -= 1; this.value3 < 0 ? this.value3 = 20 : this.value3; } } })); }); </script>
Size
Code<!-- large --> <div class="flex"> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-primary" @click="value4--;if(value4 < 0) value4 = 0;"> <svg> ... </svg> </button> <input type="number" x-model="value4" placeholder="55" class="form-input form-input-lg rounded-none text-center" min="0" max="25" readonly @wheel="changeValue($event, 'value4')" /> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-primary" @click="value4++; if(value4 > 25) value4 = 25;"> <svg> ... </svg> </button> </div> <!-- default --> <div class="flex"> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-primary" @click="value5--;if(value5 < 0) value5 = 0;"> <svg> ... </svg> </button> <input type="number" x-model="value5" placeholder="55" class="form-input rounded-none text-center" min="0" max="25" readonly @wheel="changeValue($event, 'value5')" /> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-primary" @click="value5++; if(value5 > 25) value5 = 25;"> <svg> ... </svg> </button> </div> <!-- small --> <div class="flex"> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-primary" @click="value6--;if(value6 < 0) value6 = 0;"> <svg> ... </svg> </button> <input type="number" x-model="value6" placeholder="55" class="form-input form-input-sm rounded-none text-center" min="0" max="25" readonly @wheel="changeValue($event, 'value6')" /> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-primary" @click="value6++; if(value6 > 25) value6 = 25;"> <svg> ... </svg> </button> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("touchspin", () => ({ value4: 0, value5: 0, value6: 0, changeValue(e, val) { e.preventDefault(); if (e.deltaY < 0) { if (val === 'value4') { this.value4++; this.value4 > 25 ? this.value4 = 25 : this.value4; } else if (val === 'value5') { this.value5++; this.value5 > 25 ? this.value5 = 25 : this.value5; } else if (val === 'value6') { this.value6++; this.value6 > 25 ? this.value6 = 25 : this.value6; } } else { if (val === 'value4') { this.value4--; this.value4 < 0 ? this.value4 = 0 : this.value4; } else if (val === 'value5') { this.value5--; this.value5 < 0 ? this.value5 = 0 : this.value5; } else if (val === 'value6') { this.value6--; this.value6 < 0 ? this.value6 = 0 : this.value6; } } } })); }); </script>
Inline spin button
Code<!-- inline buttons --> <div class="inline-flex"> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-primary" @click="value7--;if(value7 < 0) value7 = 0;"> <svg> ... </svg> </button> <input type="number" x-model="value7" placeholder="55" class="form-input rounded-none text-center" min="0" max="25" readonly @wheel="changeValue($event, 'value7')" /> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-primary" @click="value7++; if(value7 > 25) value7 = 25;"> <svg> ... </svg> </button> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("touchspin", () => ({ value7: 0, changeValue(e, val) { e.preventDefault(); if (e.deltaY < 0) { this.value7++; this.value7 > 25 ? this.value7 = 25 : this.value7; } else { this.value7--; this.value7 < 0 ? this.value7 = 0 : this.value7; } } })); }); </script>
Vertical spin button
Code<!-- vertical buttons --> <div class="inline-flex flex-col w-[50px]"> <button type="button" class="bg-primary text-white flex justify-center items-center rounded-t-md p-3 font-semibold border border-b-0 border-primary" @click="value8--;if(value8 < 0) value8 = 0;"> <svg> ... </svg> </button> <input type="number" x-model="value8" placeholder="55" class="form-input rounded-none text-center px-2" min="0" max="25" readonly @wheel="changeValue($event, 'value8')" /> <button type="button" class="bg-primary text-white flex justify-center items-center rounded-b-md p-3 font-semibold border border-t-0 border-primary" @click="value8++; if(value8 > 25) value8 = 25;"> <svg> ... </svg> </button> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("touchspin", () => ({ value8: 0, changeValue(e, val) { e.preventDefault(); if (e.deltaY < 0) { this.value8++; this.value8 > 25 ? this.value8 = 25 : this.value8; } else { this.value8--; this.value8 < 0 ? this.value8 = 0 : this.value8; } } })); }); </script>
Text with spin button
Code<!-- text with spin button --> <div class="flex"> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-primary" @click="value9--;if(value9 < 0) value9 = 6;"> <svg> ... </svg> </button> <input type="text" x-model="dayFormatter(value9)" placeholder="Sunday" class="form-input rounded-none text-center" min="0" max="6" readonly @wheel="changeValue($event, 'value9')" /> <button type="button" class="bg-primary text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-primary" @click="value9++; if(value9 > 6) value9 = 0;"> <svg> ... </svg> </button> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("touchspin", () => ({ value9: 0, days: ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'], dayFormatter(value) { return this.days[value]; }, changeValue(e, val) { e.preventDefault(); if (e.deltaY < 0) { this.value9++; this.value9 > 6 ? this.value9 = 0 : this.value9; } else { this.value9--; this.value9 < 0 ? this.value9 = 6 : this.value9; } } })); }); </script>
Change button class
Code<!-- basic --> <div class="flex"> <button type="button" class="bg-danger text-white flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border border-r-0 border-danger" @click="value10--;if(value10 < 0) value10 = 0;"> <svg> ... </svg> </button> <input type="number" x-model="value10" placeholder="55" class="form-input rounded-none text-center" min="0" max="25" readonly @wheel="changeValue($event, 'value10')" /> <button type="button" class="bg-warning text-white flex justify-center items-center ltr:rounded-r-md rtl:rounded-l-md px-3 font-semibold border border-l-0 border-warning" @click="value10++; if(value10 > 25) value10 = 25;"> <svg> ... </svg> </button> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("touchspin", () => ({ value10: 0, changeValue(e, val) { e.preventDefault(); if (e.deltaY < 0) { this.value10++; this.value10 > 25 ? this.value10 = 25 : this.value10; } else { this.value10--; this.value10 < 0 ? this.value10 = 0 : this.value10; } } })); }); </script>
©
. Vristo All rights reserved.