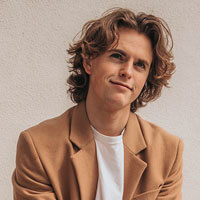
-
John DoePro
johndoe@gmail.com - Profile
- Inbox
- Lock Screen
- Sign Out
- Forms
- Basic
Input Text
Code<!-- input text --> <form> <input type="text" placeholder="Some Text..." class="form-input" required /> <button type="submit" class="btn btn-primary mt-6">Submit</button> </form>
Input Password
Code<!-- input password --> <form> <input type="password" placeholder="Enter Password" class="form-input" required /> <button type="submit" class="btn btn-primary mt-6">Submit</button> </form>
Input Email
Code<!-- input email --> <form> <input type="email" placeholder="email@mail.com" class="form-input" required /> <button type="submit" class="btn btn-primary mt-6">Submit</button> </form>
Input Url
Code<!-- input url --> <form> <input type="url" placeholder="https://dummyurl.com" class="form-input" required /> <button type="submit" class="btn btn-primary mt-6">Submit</button> </form>
Input Telephone
Code<!-- input telephone --> <form> <input type="tel" placeholder="6-(666)-111-7777" class="form-input" required /> <button type="submit" class="btn btn-primary mt-6">Submit</button> </form>
Input Search
Code<!-- input search --> <form> <input type="search" placeholder="Search..." class="form-input" required /> <button type="button" class="btn btn-primary mt-6">Submit</button> </form>
Input Range
Code<!-- input range --> <form> <input type="range" class="w-full py-2.5" min="0" max="100" /> </form>
Input With Label
Code<!-- input with label --> <form> <label for="fullname">Full Name</label> <input id="fullname" type="text" placeholder="Enter Full Name" value="Alan Green" class="form-input" /> </form>
Form controls
Code<!-- form controls --> <form class="space-y-5"> <div> <label for="ctnEmail">Email address</label> <input id="ctnEmail" type="email" placeholder="name@example.com" class="form-input" required /> </div> <div> <label for="ctnSelect1">Example select</label> <select id="ctnSelect1" class="form-select text-white-dark" required> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> </select> </div> <div> <label for="ctnSelect2">Example multiple select</label> <select id="ctnSelect2" multiple="multiple" class="form-multiselect text-white-dark" required> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> </select> </div> <div> <label for="ctnTextarea">Example textarea</label> <textarea id="ctnTextarea" rows="3" class="form-textarea" placeholder="Enter Textarea" required></textarea> </div> <div> <label for="ctnFile">Example file input</label> <input id="ctnFile" type="file" class="form-input file:py-2 file:px-4 file:border-0 file:font-semibold p-0 file:bg-primary/90 ltr:file:mr-5 rtl:file:ml-5 file:text-white file:hover:bg-primary" required /> </div> <button type="submit" class="btn btn-primary !mt-6">Submit</button> </form>
Form grid
Code<!-- form grid --> <form> <div class="grid grid-cols-1 sm:flex justify-between gap-5"> <input type="text" placeholder="Enter First Name" class="form-input" /> <input type="text" placeholder="Enter Last Name" class="form-input" /> </div> <button type="button" class="btn btn-primary mt-6">Submit</button> </form>
Form row
Code<!-- form row --> <form> <div class="grid grid-cols-1 sm:flex justify-between gap-5"> <input type="text" placeholder="Enter First Name" class="form-input" /> <input type="text" placeholder="Enter Last Name" class="form-input" /> </div> <button type="button" class="btn btn-primary mt-6">Submit</button> </form>
Form groups
Code<!-- form groups --> <form class="space-y-5"> <div> <label for="groupFname">Enter First Name</label> <input id="groupFname" type="text" placeholder="Enter First Name" class="form-input" /> </div> <div> <label for="groupLname">Enter Last Name</label> <input id="groupLname" type="text" placeholder="Enter Last Name" class="form-input" /> </div> <button type="button" class="btn btn-primary !mt-6">Submit</button> </form>
Column sizing
Code<!-- column sizing --> <form> <div class="grid grid-cols-1 md:grid-cols-3 lg:grid-cols-4 gap-2"> <input type="text" placeholder="Enter City" class="form-input lg:col-span-2" /> <input type="text" placeholder="Enter State" class="form-input" /> <input type="text" placeholder="Enter Zip" class="form-input" /> </div> <button type="button" class="btn btn-primary mt-6">Submit</button> </form>
Input with help text ( Default Left)
Code<!-- input with help text --> <form> <div> <input type="text" placeholder="Enter First Name" class="form-input" /> <span class="text-white-dark text-xs">I am the helper text.</span> </div> </form>
Input with badge help text (Default Left)
Code<!-- input with badge help text --> <form> <div> <input type="text" placeholder="Enter First Name" class="form-input mb-2" /> <span class="badge bg-primary text-xs hover:top-0">I am the helper text.</span> </div> </form>
Input with block badge help text (Default Left)
Code<!-- input with block badge help text --> <form> <div> <input type="text" placeholder="Enter First Name" class="form-input mb-2" /> <span class="badge bg-primary block text-xs hover:top-0">I am the helper text.</span> </div> </form>
Inline Help text
Code<!-- inline Help text --> <form> <div> <label for="Txtpassword">Password</label> <input id="Txtpassword" type="password" placeholder="Enter Password" class="form-input w-3/5" /> <span class="text-xs text-white-dark ltr:pl-2 rtl:pr-2">Min 8-20 char</span> </div> </form>
Input Fields
Code<!-- input fields --> <form> <div class="grid grid-cols-1 sm:grid-cols-3 gap-4"> <div> <label for="inputLarge">Large Input</label> <input id="inputLarge" type="text" placeholder="Large Input" class="form-input form-input-lg" /> </div> <div> <label for="inputDefault">Default Input</label> <input id="inputDefault" type="text" placeholder="Default Input" class="form-input" /> </div> <div> <label for="inputSmall">Small Input</label> <input id="inputSmall" type="text" placeholder="Small Input" class="form-input form-input-sm" /> </div> </div> </form>
Select Field
Code<!-- select field --> <form> <div class="grid grid-cols-1 sm:grid-cols-3 gap-4"> <div> <select class="form-select form-select-lg text-white-dark"> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> </select> </div> <div> <select class="form-select text-white-dark"> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> </select> </div> <div> <select class="form-select form-select-sm text-white-dark"> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> </select> </div> </div> </form>
Horizontal form label sizing
Code<!-- horizontal form label sizing --> <form class="space-y-5"> <div class="sm:flex justify-between items-center gap-5 md:gap-20"> <label for="hrLargeinput">Email</label> <input id="hrLargeinput" type="email" placeholder="name@example.com" class="form-input py-2.5 text-base" /> </div> <div class="sm:flex justify-between items-center gap-5 md:gap-20"> <label for="hrDefaultinput">Email</label> <input id="hrDefaultinput" type="email" placeholder="name@example.com" class="form-input" /> </div> <div class="sm:flex justify-between items-center gap-5 md:gap-20"> <label for="hrSmallinput">Email</label> <input for="hrSmallinput" type="email" placeholder="name@example.com" class="form-input py-1.5 text-xs" /> </div> </form>
Input Readonly
Code<!-- input readonly --> <form> <div> <input type="text" placeholder="Readonly input here…" class="form-input disabled:pointer-events-none" readonly /> </div> </form>
Disabled Fields
Code<!-- disabled fileds --> <form class="space-y-5"> <div> <label for="disInput" class="text-white-dark">Disabled input</label> <input id="disInput" type="text" placeholder="Readonly input here…" class="form-input disabled:pointer-events-none disabled:bg-[#eee] dark:disabled:bg-[#1b2e4b] cursor-not-allowed" disabled /> </div> <div> <label for="disSelect" class="text-white-dark">Disabled select menu</label> <select id="disSelect" class="form-select disabled:pointer-events-none disabled:bg-[#eee] dark:disabled:bg-[#1b2e4b] text-white-dark" disabled> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> </select> </div> <div> <label class="flex items-center"> <input type="checkbox" class="form-checkbox" disabled /> <span class="text-white-dark"">Can't check this</span> </label> </div> <button type="submit" class="btn btn-primary !mt-6 disabled:pointer-events-none disabled:opacity-60" disabled>Submit</button> </form>
Checkboxes
Code<!-- checkboxes --> <form> <div> <label class="flex items-center cursor-pointer"> <input type="checkbox" class="form-checkbox" checked /> <span class=" text-white-dark"">Checkbox</span> </label> </div> </form>
Radio
Code<!-- radio --> <form class="space-y-5"> <div> <label class="flex items-center cursor-pointer"> <input type="radio" name="custom_radio2" class="form-radio" checked /> <span class="text-white-dark"">Toggle this custom radio</span> </label> </div> <div> <label class="flex items-center cursor-pointer"> <input type="radio" name="custom_radio2" class="form-radio" /> <span class="text-white-dark"">Or toggle this other custom radio</span> </label> </div> </form>
Disabled
Code<!-- disabled --> <form class="space-y-5"> <div> <label class="flex items-center"> <input type="checkbox" class="form-checkbox" disabled /> <span class=" text-white-dark"">Check this custom checkbox</span> </label> </div> <div> <label class="flex items-center"> <input type="radio" class="form-radio" disabled /> <span class="text-white-dark"">Toggle this custom radio</span> </label> </div> </form>
Select menu
Code<!-- select menu --> <form> <div> <select class="form-select text-white-dark"> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> </select> </div> </form>
Multiselect
Code<!-- multiselect --> <form> <div> <select size="4" multiple="multiple" class="form-multiselect text-white-dark !bg-none"> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> <option>Four</option> <option>Five</option> </select> </div> </form>
©
. Vristo All rights reserved.