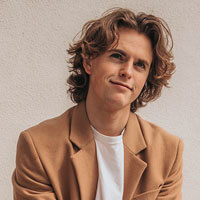
-
John DoePro
johndoe@gmail.com - Profile
- Inbox
- Lock Screen
- Sign Out
- Components
- Sweet Alerts
Basic message
Code<!-- basic message --> <button type="button" class="btn btn-primary" @click="showAlert()">Basic message</button> <!-- script --> <script> async function showAlert() { new window.Swal({ title: 'Saved succesfully', padding: '2em', }); } </script>
Success message
Code<!-- success message --> <button type="button" class="btn btn-secondary" @click="showAlert()">Success message!</button> <!-- script --> <script> async function showAlert() { new window.Swal({ icon: 'success', title: 'Good job!', text: 'You clicked the!', padding: '2em', }); } </script>
Dynamic queue
Code<!-- dynamic queue --> <button type="button" class="btn btn-success" @click="showAlert()">Dynamic queue</button> <!-- script --> <script> async function showAlert() { const ipAPI = 'https://api.ipify.org?format=json'; new window.Swal({ title: 'Your public IP', confirmButtonText: 'Show my public IP', text: 'Your public IP will be received ' + 'via AJAX request', showLoaderOnConfirm: true, preConfirm: () => { return fetch(ipAPI) .then((response) => { return response.json(); }) .then((data) => { new window.Swal({ title: data.ip, }); }) .catch(() => { new window.Swal({ type: 'error', title: 'Unable to get your public IP', }); }); }, }); } </script>
A title with a text under
Code<!-- success message --> <button type="button" class="btn btn-danger" @click="showAlert()">Title & text</button> <!-- script --> <script> async function showAlert() { new window.Swal({ icon: 'question', title: 'The Internet?', text: 'That thing is still around?', padding: '2em', }); } </script>
Chaining modals (queue)
Code<!-- success message --> <button type="button" class="btn btn-warning" @click="showAlert()">Chaining modals (queue)</button> <!-- script --> <script> async function showAlert() { const steps = ['1', '2', '3']; const swalQueueStep = window.Swal.mixin({ confirmButtonText: 'Next →', showCancelButton: true, progressSteps: steps, input: 'text', inputAttributes: { required: true, }, validationMessage: 'This field is required', padding: '2em', }); const values = []; let currentStep; for (currentStep = 0; currentStep < steps.length;) { const result = await swalQueueStep.fire({ title: `Question ${steps[currentStep]}`, text: currentStep == 0 ? 'Chaining swal modals is easy.' : '', inputValue: values[currentStep], showCancelButton: currentStep > 0, currentProgressStep: currentStep, }); if (result.value) { values[currentStep] = result.value; currentStep++; } else if (result.dismiss === Swal.DismissReason.cancel) { currentStep--; } else { break; } } if (currentStep === steps.length) { window.Swal.fire({ title: 'All done!', padding: '2em', html: 'Your answers: <pre>' + JSON.stringify(values) + '</pre>', confirmButtonText: 'Lovely!', }); } } else if (type === 6) { new window.Swal({ title: 'Custom animation with Animate.css', animation: false, showClass: { popup: 'animate__animated animate__flip' }, hideClass: { popup: 'animate__animated animate__fadeOutUp' }, padding: '2em', }); } </script>
Custom animation
Code<!-- success message --> <button type="button" class="btn btn-info" @click="showAlert()">Custom animation</button> <!-- script --> <script> async function showAlert() { new window.Swal({ title: 'Custom animation with Animate.css', animation: false, showClass: { popup: 'animate__animated animate__flip' }, hideClass: { popup: 'animate__animated animate__fadeOutUp' }, padding: '2em', }); } </script>
Message with auto close timer
Code<!-- success message --> <button type="button" class="btn btn-primary" @click="showAlert()">Message timer</button> <!-- script --> <script> async function showAlert() { let timerInterval; new window.Swal({ title: 'Auto close alert!', html: 'I will close in <b></b> milliseconds.', timer: 2000, timerProgressBar: true, didOpen: () => { window.Swal.showLoading(); const b = window.Swal.getHtmlContainer().querySelector('b'); timerInterval = setInterval(() => { b.textContent = window.Swal.getTimerLeft(); }, 100); }, willClose: () => { clearInterval(timerInterval); }, }).then((result) => { if (result.dismiss === window.Swal.DismissReason.timer) { console.log('I was closed by the timer'); } }); } </script>
Message with custom image
Code<!-- success message --> <button type="button" class="btn btn-secondary" @click="showAlert()">Message with custom image</button> <!-- script --> <script> async function showAlert() { new window.Swal({ title: 'Sweet!', text: 'Modal with a custom image.', imageUrl: ('assets/images/thumbs-up.jpg'), imageWidth: 224, imageHeight: 200, imageAlt: 'Custom image', animation: false, padding: '2em', }); } </script>
Custom HTML description and buttons
Code<!-- success message --> <button type="button" class="btn btn-danger" @click="showAlert()">Custom Description & buttons</button> <!-- script --> <script> async function showAlert() { new window.Swal({ icon: 'info', title: '<i>HTML</i> <u>example</u>', html: 'You can use <b>bold text</b>, ' + '<a href="//github.com">links</a> ' + 'and other HTML tags', showCloseButton: true, showCancelButton: true, focusConfirm: false, confirmButtonText: '<i class="flaticon-checked-1"></i> Great!', confirmButtonAriaLabel: 'Thumbs up, great!', cancelButtonText: '<i class="flaticon-cancel-circle"></i> Cancel', cancelButtonAriaLabel: 'Thumbs down', padding: '2em', }); } </script>
Warning message, with "Confirm" button
Code<!-- success message --> <button type="button" class="btn btn-success" @click="showAlert()">Confirm</button> <!-- script --> <script> async function showAlert() { new window.Swal({ icon: 'warning', title: 'Are you sure?', text: "You won't be able to revert this!", showCancelButton: true, confirmButtonText: 'Delete', padding: '2em', }).then((result) => { if (result.value) { new window.Swal('Deleted!', 'Your file has been deleted.', 'success'); } }); } </script>
Execute something else for "Cancel".
Code<!-- success message --> <button type="button" class="btn btn-warning" @click="showAlert()">Addition else for "Cancel".</button> <!-- script --> <script> async function showAlert() { const swalWithBootstrapButtons = window.Swal.mixin({ confirmButtonClass: 'btn btn-secondary', cancelButtonClass: 'btn btn-dark ltr:mr-3 rtl:ml-3', buttonsStyling: false, }); swalWithBootstrapButtons .fire({ title: 'Are you sure?', text: "You won't be able to revert this!", icon: 'warning', showCancelButton: true, confirmButtonText: 'Yes, delete it!', cancelButtonText: 'No, cancel!', reverseButtons: true, padding: '2em', }) .then((result) => { if (result.value) { swalWithBootstrapButtons.fire('Deleted!', 'Your file has been deleted.', 'success'); } else if (result.dismiss === window.Swal.DismissReason.cancel) { swalWithBootstrapButtons.fire('Cancelled', 'Your imaginary file is safe :)', 'error'); } }); } </script>
A message with custom width, padding and background
Code<!-- success message --> <button type="button" class="btn btn-info" @click="showAlert()">Custom Message</button> <!-- script --> <script> async function showAlert() { new window.Swal({ title: 'Custom width, padding, background.', width: 600, padding: '7em', customClass: 'background-modal', background: '#fff url(' + ('assets/images/sweet-bg.jpg') + ') no-repeat 100% 100%', }); } </script>
With Footer
Code<!-- success message --> <button type="button" class="btn btn-dark" @click="showAlert()">With Footer</button> <!-- script --> <script> async function showAlert() { new window.Swal({ icon: 'error', title: 'Oops...', text: 'Something went wrong!', footer: '<a href="javascript:;">Why do I have this issue?</a>', padding: '2em', }); } </script>
RTL support
Code<!-- success message --> <button type="button" class="btn btn-primary" @click="showAlert()">RTL</button> <!-- script --> <script> async function showAlert() { new window.Swal({ title: 'هل تريد الاستمرار؟', confirmButtonText: 'نعم', cancelButtonText: 'لا', showCancelButton: true, showCloseButton: true, padding: '2em', }); } </script>
Mixin
Code<!-- success message --> <button type="button" class="btn btn-secondary" @click="showAlert()">Mixin</button> <!-- script --> <script> async function showAlert() { const toast = window.Swal.mixin({ toast: true, position: 'top-end', showConfirmButton: false, timer: 3000, padding: '2em', }); toast.fire({ icon: 'success', title: 'Signed in successfully', padding: '2em', }); } </script>
©
. Vristo All rights reserved.