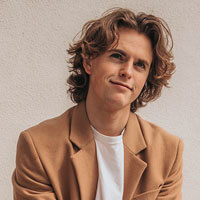
-
John DoePro
johndoe@gmail.com - Profile
- Inbox
- Lock Screen
- Sign Out
- Components
- Notifications
Basic
Code<!-- basic --> <button type="button" class="btn btn-primary" @click="showMessage('Hello, world! This is a toast message.')">Open Toast</button> <!-- script --> <script> showMessage = (msg = 'Example notification text.', position = 'bottom-start', showCloseButton = true, closeButtonHtml = '', duration = 3000) => { const toast = window.Swal.mixin({ toast: true, position: position || 'bottom-start', showConfirmButton: false, timer: duration, showCloseButton: showCloseButton, }); toast.fire({ title: msg, }); }; </script>
Position
CodeTop Position
Bottom Position
<!-- top left --> <button type="button" class="btn btn-success" @click="showMessage('Example notification text.',$store.app.rtlClass === 'rtl' ? 'top-end' : 'top-start')">Top Left</button> <!-- top center --> <button type="button" class="btn btn-secondary" @click="showMessage('Example notification text','top')">Top Center</button> <!-- top right --> <button type="button" class="btn btn-info" @click="showMessage('Example notification text.', $store.app.rtlClass === 'rtl' ? 'top-start' : 'top-end')">Top Right</button> <!-- bottom left --> <button type="button" class="btn btn-dark" @click="showMessage('Example notification text.',$store.app.rtlClass === 'rtl' ? 'bottom-end' : 'bottom-start')">Bottom Left</button> <!-- bottom center --> <button type="button" class="btn btn-primary" @click="showMessage('Example notification text.','bottom')">Bottom Center</button> <!-- bottom right --> <button type="button" class="btn btn-secondary" @click="showMessage('Example notification text.',$store.app.rtlClass === 'rtl' ? 'bottom-start' : 'bottom-end')">Bottom Right</button> <!-- script --> <script> showMessage = (msg = 'Example notification text.', position = 'bottom-start', showCloseButton = true, closeButtonHtml = '', duration = 3000) => { const toast = window.Swal.mixin({ toast: true, position: position || 'bottom-start', showConfirmButton: false, timer: duration, showCloseButton: showCloseButton, }); toast.fire({ title: msg, }); }; </script>
No Action
Code<!-- no action --> <button type="button" class="btn btn-success" @click="showMessage('Example notification text.', 'bottom-start',false)">No Action</button> <!-- script --> <script> showMessage = (msg = 'Example notification text.', position = 'bottom-start', showCloseButton = true, closeButtonHtml = '', duration = 3000) => { const toast = window.Swal.mixin({ toast: true, position: position || 'bottom-start', showConfirmButton: false, timer: duration, showCloseButton: showCloseButton, }); toast.fire({ title: msg, }); }; </script>
Click Callback
Code<!-- click callback --> <button type="button" class="btn btn-info" @click="clickCallable()">Click Callback</button> <!-- script --> <script> clickCallable = () => { new window.Swal({ toast: true, position: 'bottom-start', text: "Custom callback when action button is clicked.", showCloseButton: true, showConfirmButton: false, // confirmButtonText: 'Delete', }).then((result) => { new window.Swal({ toast: true, position: 'bottom-start', text: 'Thanks for clicking the Dismiss button!', showCloseButton: true, showConfirmButton: false, }); }); }; </script>
Duration
Code<!-- click callback --> <button type="button" class="btn btn-dark" @click="showMessage(msg='Example notification text.', position= 'bottom-start', duration=5000)">Duration</button> <!-- script --> <script> showMessage = (msg = 'Example notification text.', position = 'bottom-start', showCloseButton = true, closeButtonHtml = '', duration = 3000) => { const toast = window.Swal.mixin({ toast: true, position: position || 'bottom-start', showConfirmButton: false, timer: duration, showCloseButton: showCloseButton, }); toast.fire({ title: msg, }); }; </script>
Background Color
Code<!-- primary --> <div> <button type="button" class="btn btn-primary" @click="coloredToast('primary')">Primary</button> <div id="primary-toast"></div> </div> <!-- secondary --> <div> <button type=" button" class="btn btn-secondary" @click="coloredToast('secondary')">Secondary</button> <div id="secondary-toast"></div> </div> <!-- success --> <div> <button type="button" class="btn btn-success" @click="coloredToast('success')">Success</button> <div id="success-toast"></div> </div> <!-- danger --> <div> <button type="button" class="btn btn-danger" @click="coloredToast('danger')">Danger</button> <div id="danger-toast"></div> </div> <!-- warning --> <div> <button type="button" class="btn btn-warning" @click="coloredToast('warning')">Warning</button> <div id="warning-toast"></div> </div> <!-- info --> <div> <button type="button" class="btn btn-info" @click="coloredToast('info')">Info</button> <div id="info-toast"></div> </div> <!-- script --> <script> coloredToast = (color) => { const toast = window.Swal.mixin({ toast: true, position: 'bottom-start', showConfirmButton: false, timer: 3000, showCloseButton: true, animation: false, customClass: { popup: `color-${color}` }, target: document.getElementById(color + '-toast') }); toast.fire({ title: 'Example notification text.', }); }; </script>
©
. Vristo All rights reserved.