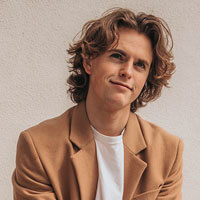
-
John DoePro
johndoe@gmail.com - Profile
- Inbox
- Lock Screen
- Sign Out
- Forms
- Validation
Basic
Code<!-- basic --> <div x-data="form"> <form class="space-y-5" @submit.prevent="submitForm1()"> <div :class="[isSubmitForm1 ? (form1.name ? 'has-success' : 'has-error') : '']"> <label for="fullName">Full Name</label> <input id="fullName" type="text" placeholder="Enter Full Name" class="form-input" x-model="form1.name" /> <template x-if="isSubmitForm1 && form1.name"> <p class="text-[#1abc9c] mt-1">Looks Good!</p> </template> <template x-if="isSubmitForm1 && !form1.name"> <p class="text-danger mt-1">Please fill the Name</p> </template> </div> <button type="submit" class="btn btn-primary !mt-6">Submit Form</button> </form> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ form1: { name: '' }, isSubmitForm1: false, submitForm1() { this.isSubmitForm1 = true; if (this.name) { //form validated success this.showMessage('Form submitted successfully.'); } }, showMessage(msg = '', type = 'success') { const toast = window.Swal.mixin({ toast: true, position: 'top', showConfirmButton: false, timer: 3000 }); toast.fire({ icon: type, title: msg, padding: '10px 20px' }); }, })); }); </script>
<!-- email --> <div x-data="form"> <form class="space-y-5" @submit.prevent="submitForm2()"> <div :class="[isSubmitForm2 ? (form2.email && emailValidate(form2.email) ? 'has-success' : 'has-error') : '']"> <label for="Email">Email</label> <input id="Email" type="text" placeholder="Enter Email" class="form-input" x-model="form2.email" /> <template x-if="isSubmitForm2 && form2.email && emailValidate(form2.email)"> <p class="text-[#1abc9c] mt-1">Looks Good!</p> </template> <template x-if="isSubmitForm2 && !(form2.email && emailValidate(form2.email))"> <p class="text-danger mt-1">Please fill the Email</p> </template> </div> <button type="submit" class="btn btn-primary !mt-6">Submit Form</button> </form> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ form2: { email: '' }, isSubmitForm2: false, emailValidate(email) { const regexp = /^[\w.%+-]+@[\w.-]+\.[\w]{2,6}$/; return regexp.test(email); }, submitForm2() { this.isSubmitForm2 = true; if (this.emailValidate(this.form2.email)) { //form validated success this.showMessage('Form submitted successfully.'); } }, showMessage(msg = '', type = 'success') { const toast = window.Swal.mixin({ toast: true, position: 'top', showConfirmButton: false, timer: 3000 }); toast.fire({ icon: type, title: msg, padding: '10px 20px' }); }, })); }); </script>
Select
Code<!-- select --> <div x-data="form"> <form class="space-y-5" @submit.prevent="submitForm3()"> <div :class="[isSubmitForm3 ? (form3.select ? 'has-success' : 'has-error') : '']"> <select class="form-select text-white-dark" x-model="form3.select"> <option>Open this select menu</option> <option>One</option> <option>Two</option> <option>Three</option> </select> <template x-if="isSubmitForm3 && form3.select"> <p class="text-[#1abc9c] mt-1">Example valid custom select feedback</p> </template> <template x-if="isSubmitForm3 && !form3.select"> <p class="text-danger mt-1">Please Select the field</p> </template> </div> <button type="submit" class="btn btn-primary !mt-6">Submit Form</button> </form> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ form3: { select: '' }, isSubmitForm3: false, submitForm3() { this.isSubmitForm3 = true; if (this.form3.select) { //form validated success this.showMessage('Form submitted successfully.'); } }, showMessage(msg = '', type = 'success') { const toast = window.Swal.mixin({ toast: true, position: 'top', showConfirmButton: false, timer: 3000 }); toast.fire({ icon: type, title: msg, padding: '10px 20px' }); }, })); }); </script>
Custom Styles
Code<!-- custom styles --> <form class="space-y-5" @submit.prevent="submitForm4()"> <div class="grid grid-cols-1 md:grid-cols-3 gap-5"> <div :class="[isSubmitForm4 ? (form4.firstName ? 'has-success' : 'has-error') : '']"> <label for="customFname">First Name</label> <input id="customFname" type="text" placeholder="Enter First Name" class="form-input" x-model="form4.firstName" /> <template x-if="isSubmitForm4 && form4.firstName"> <p class="text-[#1abc9c] mt-1">Looks Good!</p> </template> <template x-if="isSubmitForm4 && !form4.firstName"> <p class="text-danger mt-1">Please fill the first name</p> </template> </div> <div :class="[isSubmitForm4 ? (form4.lastName ? 'has-success' : 'has-error') : '']"> <label for="customLname">Last name</label> <input id="customLname" type="text" placeholder="Enter Last Name" class="form-input" x-model="form4.lastName" /> <template x-if="isSubmitForm4 && form4.lastName"> <p class="text-[#1abc9c] mt-1">Looks Good!</p> </template> <template x-if="isSubmitForm4 && !form4.lastName"> <p class="text-danger mt-1">Please fill the last name</p> </template> </div> <div :class="[isSubmitForm4 ? (form4.userName ? 'has-success' : 'has-error') : '']"> <label for="customeEmail">Username</label> <div class="flex"> <div class="bg-[#eee] flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border ltr:border-r-0 rtl:border-l-0 border-[#e0e6ed] dark:border-[#17263c] dark:bg-[#1b2e4b]">@</div> <input id="customeEmail" type="text" placeholder="Enter Username" class="form-input ltr:rounded-l-none rtl:rounded-r-none" x-model="form4.userName" /> </div> <template x-if="isSubmitForm4 && form4.userName"> <p class="text-[#1abc9c] mt-1">Looks Good!</p> </template> <template x-if="isSubmitForm4 && !form4.userName"> <p class="text-danger mt-1">Please choose a userName</p> </template> </div> </div> <div class="grid grid-cols-1 md:grid-cols-4 gap-5"> <div class="md:col-span-2" :class="[isSubmitForm4 ? (form4.city ? 'has-success' : 'has-error') : '']"> <label for="customeCity">City</label> <input id="customeCity" type="text" placeholder="Enter City" class="form-input" x-model="form4.city" /> <template x-if="isSubmitForm4 && form4.city"> <p class="text-[#1abc9c] mt-1">Looks Good!</p> </template> <template x-if="isSubmitForm4 && !form4.city"> <p class="text-danger mt-1">Please provide a valid city</p> </template> </div> <div :class="[isSubmitForm4 ? (form4.state ? 'has-success' : 'has-error') : '']"> <label for="customeState">State</label> <input id="customeState" type="text" placeholder="Enter State" class="form-input" x-model="form4.state" /> <template x-if="isSubmitForm4 && form4.state"> <p class="text-[#1abc9c] mt-1">Looks Good!</p> </template> <template x-if="isSubmitForm4 && !form4.state"> <p class="text-danger mt-1">Please provide a valid state</p> </template> </div> <div :class="[isSubmitForm4 ? (form4.zip ? 'has-success' : 'has-error') : '']"> <label for="customeZip">Zip</label> <input id="customeZip" type="text" placeholder="Enter Zip" class="form-input" x-model="form4.zip" /> <template x-if="isSubmitForm4 && form4.zip"> <p class="text-[#1abc9c] mt-1">Looks Good!</p> </template> <template x-if="isSubmitForm4 && !form4.zip"> <p class="text-danger mt-1">Please provide a valid zip</p> </template> </div> </div> <div :class="[isSubmitForm4 ? (form4.isTerms ? 'has-success' : 'has-error') : '']"> <label class="inline-flex cursor-pointer mt-1"> <input type="checkbox" class="form-checkbox" x-model="form4.isTerms" /> <span class="text-white-dark" ">Agree to terms and conditions</span> </label> <template x-if="isSubmitForm4 && !form4.isTerms"> <p class="text-danger mt-1">You must agree before submitting.</p> </template> </div> <button type="submit" class="btn btn-primary !mt-6">Submit Form</button> </form> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ form4: { firstName: 'Shaun', lastName: 'Park', userName: '', city: '', state: '', zip: '', isTerms: false }, isSubmitForm4: false, submitForm4() { this.isSubmitForm4 = true; if (this.form4.firstName && this.form4.lastName && this.form4.userName && this.form4.city && this.form4.state && this.form4.zip && this.form4.isTerms) { //form validated success this.showMessage('Form submitted successfully.'); } }, showMessage(msg = '', type = 'success') { const toast = window.Swal.mixin({ toast: true, position: 'top', showConfirmButton: false, timer: 3000 }); toast.fire({ icon: type, title: msg, padding: '10px 20px' }); }, })); }); </script>
Browser Default
Code<!-- browser default --> <div x-data="form"> <form class="space-y-5" @submit.prevent="submitForm5()"> <div class="grid grid-cols-1 md:grid-cols-3 gap-5"> <div> <label for="browserFname">First Name</label> <input id="browserFname" type="text" placeholder="Enter First Name" x-model="form5.firstName" class="form-input" required /> </div> <div> <label for="browserLname">Last name</label> <input id="browserLname" type="text" placeholder="Enter Last name" x-model="form5.lastName" class="form-input" required /> </div> <div> <label for="browserEmail">Username</label> <div class="flex"> <div class="bg-[#eee] flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border ltr:border-r-0 rtl:border-l-0 border-[#e0e6ed] dark:border-[#17263c] dark:bg-[#1b2e4b]">@</div> <input id="browserEmail" type="text" placeholder="Enter Username" x-model="form5.userName" class="form-input ltr:rounded-l-none rtl:rounded-r-none" required /> </div> </div> </div> <div class="grid grid-cols-1 md:grid-cols-4 gap-5"> <div class="md:col-span-2"> <label for="browserCity">City</label> <input id="browserCity" type="text" placeholder="Enter City" x-model="form5.city" class="form-input" required /> </div> <div> <label for="browserState">State</label> <input id="browserState" type="text" placeholder="Enter State" x-model="form5.state" class="form-input" required /> </div> <div> <label for="browserZip">Zip</label> <input id="browserZip" type="text" placeholder="Enter Zip" x-model="form5.zip" class="form-input" required /> </div> </div> <div> <label class="flex items-center cursor-pointer mt-1"> <input type="checkbox" class="form-checkbox" x-model="form5.isTerms" require /> <span class="text-white-dark"">Agree to terms and conditions</span> </label> </div> <button type="submit" class="btn btn-primary !mt-6">Submit Form</button> </form> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ form5: { firstName: 'Shaun', lastName: 'Park', userName: '', city: '', state: '', zip: '', isTerms: false }, isSubmitForm5: false, submitForm5() { this.isSubmitForm5 = true; if (this.form5.firstName && this.form5.lastName && this.form5.userName && this.form5.city && this.form5.state && this.form5.zip && this.form5.isTerms) { //form validated success this.showMessage('Form submitted successfully.'); } }, showMessage(msg = '', type = 'success') { const toast = window.Swal.mixin({ toast: true, position: 'top', showConfirmButton: false, timer: 3000 }); toast.fire({ icon: type, title: msg, padding: '10px 20px' }); }, })); }); </script>
Tooltips
Code<!-- tooltips --> <div x-data="form"> <form class="space-y-5" @submit.prevent="submitForm6()"> <div class="grid grid-cols-1 md:grid-cols-3 gap-5"> <div :class="[isSubmitForm6 ? (form6.firstName ? 'has-success' : 'has-error') : '']"> <label for="tlpFname">First Name</label> <input id="tlpFname" type="text" placeholder="Enter First Name" class="form-input mb-2" x-model="form6.firstName" /> <template x-if="isSubmitForm6 && form6.firstName"> <span class="text-white bg-[#1abc9c] py-1 px-2 rounded">Looks Good!</span> </template> <template x-if="isSubmitForm6 && !form6.firstName"> <span class="text-white bg-danger py-1 px-2 rounded">Please fill the first Name</span> </template> </div> <div :class="[isSubmitForm6 ? (form6.lastName ? 'has-success' : 'has-error') : '']"> <label for="tlpLname">Last name</label> <input id="tlpLname" type="text" placeholder="Enter Last Name" class="form-input mb-2" x-model="form6.lastName" /> <template x-if="isSubmitForm6 && form6.lastName"> <span class="text-white bg-[#1abc9c] py-1 px-2 rounded">Looks Good!</span> </template> <template x-if="isSubmitForm6 && !form6.lastName"> <span class="text-white bg-danger py-1 px-2 rounded">Please fill the last Name</span> </template> </div> <div :class="[isSubmitForm6 ? (form6.userName ? 'has-success' : 'has-error') : '']"> <label for="tlpEmail">Username</label> <div class="flex"> <div class="bg-[#eee] flex justify-center items-center ltr:rounded-l-md rtl:rounded-r-md px-3 font-semibold border ltr:border-r-0 rtl:border-l-0 border-[#e0e6ed] dark:border-[#17263c] dark:bg-[#1b2e4b]">@</div> <input id="tlpEmail" type="text" placeholder="Enter Username" class="form-input ltr:rounded-l-none rtl:rounded-r-none" x-model="form6.userName" /> </div> <div class="mt-2"> <template x-if="isSubmitForm6 && form6.userName"> <span class="text-white bg-[#1abc9c] py-1 px-2 rounded">Looks Good!</span> </template> <template x-if="isSubmitForm6 && !form6.userName"> <span class="text-white bg-danger py-1 px-2 rounded">Please choose a userName.</span> </template> </div> </div> </div> <div class="grid grid-cols-1 md:grid-cols-4 gap-5"> <div class="md:col-span-2" :class="[isSubmitForm6 ? (form6.city ? 'has-success' : 'has-error') : '']"> <label for="tlpCity">City</label> <input id="tlpCity" type="text" placeholder="Enter City" class="form-input mb-2" x-model="form6.city" /> <template x-if="isSubmitForm6 && form6.city"> <span class="text-white bg-[#1abc9c] py-1 px-2 rounded">Looks Good!</span> </template> <template x-if="isSubmitForm6 && !form6.city"> <span class="text-white bg-danger py-1 px-2 rounded">Please provide a valid city.</span> </template> </div> <div :class="[isSubmitForm6 ? (form6.state ? 'has-success' : 'has-error') : '']"> <label for="tlpState">State</label> <input id="tlpState" type="text" placeholder="Enter State" class="form-input mb-2" x-model="form6.state" /> <template x-if="isSubmitForm6 && form6.state"> <span class="text-white bg-[#1abc9c] py-1 px-2 rounded">Looks Good!</span> </template> <template x-if="isSubmitForm6 && !form6.state"> <span class="text-white bg-danger py-1 px-2 rounded">Please provide a valid state.</span> </template> </div> <div :class="[isSubmitForm6 ? (form6.zip ? 'has-success' : 'has-error') : '']"> <label for="tlpZip">Zip</label> <input id="tlpZip" type="text" placeholder="Enter Zip" class="form-input mb-2" x-model="form6.zip" /> <template x-if="isSubmitForm6 && form6.zip"> <span class="text-white bg-[#1abc9c] py-1 px-2 rounded">Looks Good!</span> </template> <template x-if="isSubmitForm6 && !form6.zip"> <span class="text-white bg-danger py-1 px-2 rounded">Please provide a valid Zip.</span> </template> </div> </div> <div :class="[isSubmitForm6 ? (form6.isTerms ? 'has-success' : 'has-error') : '']"> <label class="flex items-center cursor-pointer"> <input type="checkbox" class="form-checkbox" x-model="form6.isTerms" /> <span class="text-white-dark"">Agree to terms and conditions</span> </label> <template x-if="isSubmitForm6 && !form6.isTerms"> <div class="mt-2"> <span class="text-white bg-danger py-1 px-2 rounded">You must agree before submitting.</span> </div> </template> </div> <button type="submit" class="btn btn-primary !mt-6">Submit Form</button> </form> <div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("form", () => ({ form6: { firstName: 'Shaun', lastName: 'Park', userName: '', city: '', state: '', zip: '', isTerms: false }, isSubmitForm6: false, submitForm6() { this.isSubmitForm6 = true; if (this.form6.firstName && this.form6.lastName && this.form6.userName && this.form6.city && this.form6.state && this.form6.zip && this.form6.isTerms) { //form validated success this.showMessage('Form submitted successfully.'); } }, showMessage(msg = '', type = 'success') { const toast = window.Swal.mixin({ toast: true, position: 'top', showConfirmButton: false, timer: 3000 }); toast.fire({ icon: type, title: msg, padding: '10px 20px' }); }, })); }); </script>
©
. Vristo All rights reserved.