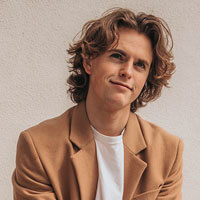
-
John DoePro
johndoe@gmail.com - Profile
- Inbox
- Lock Screen
- Sign Out
- Components
- Countdown
Simple Countdown
CodeDays
Hours
Mins
Sec
<!-- simple countdown --> <div x-data="countdown"></div> <div class="mb-5 grid grid-cols-4 justify-items-center gap-3" x-init="setTimerDemo1"> <div> <div class="w-16 h-16 sm:w-[100px] sm:h-[100px] shadow-[1px_2px_12px_0_rgba(31,45,61,0.10)] rounded border border-[#e0e6ed] dark:border-[#1b2e4b] flex justify-center flex-col"> <h1 class="text-primary sm:text-3xl text-xl text-center" x-text="demo1.days"></h1> </div> <h4 class="text-[#3b3f5c] text-[15px] mt-4 text-center dark:text-white-dark font-semibold">Days</h4> </div> <div> <div class="w-16 h-16 sm:w-[100px] sm:h-[100px] shadow-[1px_2px_12px_0_rgba(31,45,61,0.10)] rounded border border-[#e0e6ed] dark:border-[#1b2e4b] flex justify-center flex-col"> <h1 class="text-primary sm:text-3xl text-xl text-center" x-text="demo1.hours"></h1> </div> <h4 class="text-[#3b3f5c] text-[15px] mt-4 text-center dark:text-white-dark font-semibold">Hours</h4> </div> <div> <div class="w-16 h-16 sm:w-[100px] sm:h-[100px] shadow-[1px_2px_12px_0_rgba(31,45,61,0.10)] rounded border border-[#e0e6ed] dark:border-[#1b2e4b] flex justify-center flex-col"> <h1 class="text-primary sm:text-3xl text-xl text-center" x-text="demo1.minutes"></h1> </div> <h4 class="text-[#3b3f5c] text-[15px] mt-4 text-center dark:text-white-dark font-semibold">Mins</h4> </div> <div> <div class="w-16 h-16 sm:w-[100px] sm:h-[100px] shadow-[1px_2px_12px_0_rgba(31,45,61,0.10)] rounded border border-[#e0e6ed] dark:border-[#1b2e4b] flex justify-center flex-col"> <h1 class="text-primary sm:text-3xl text-xl text-center" x-text="demo1.seconds"></h1> </div> <h4 class="text-[#3b3f5c] text-[15px] mt-4 text-center dark:text-white-dark font-semibold">Sec</h4> </div> </div> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("countdown", () => ({ timer1: null, demo1: { days: null, hours: null, minutes: null, seconds: null, }, setTimerDemo1() { let date = new Date(); date.setDate(date.getDate() + 3); let countDownDate = date.getTime(); timer1 = setInterval(() => { let now = new Date().getTime(); let distance = countDownDate - now; this.demo1.days = Math.floor(distance / (1000 * 60 * 60 * 24)); this.demo1.hours = Math.floor((distance % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)); this.demo1.minutes = Math.floor((distance % (1000 * 60 * 60)) / (1000 * 60)); this.demo1.seconds = Math.floor((distance % (1000 * 60)) / 1000); if (distance < 0) { clearInterval(this.timer1); } }, 500); }, }); }); </script>
Circle Countdown
CodeDays
Hours
Mins
Sec
<!-- circle countdown --> <div x-data="countdown"> <div class="mb-5 grid grid-cols-4 justify-items-center gap-3" x-init="setTimerDemo2"> <div> <div class="w-16 h-16 sm:w-[100px] sm:h-[100px] shadow-[1px_2px_12px_0_rgba(31,45,61,0.10)] rounded-full border border-[#e0e6ed] dark:border-[#1b2e4b] flex justify-center flex-col"> <h1 class="text-primary sm:text-3xl text-xl text-center" x-text="demo2.days"></h1> </div> <h4 class="text-[#3b3f5c] text-[15px] mt-4 text-center dark:text-white-dark font-semibold">Days</h4> </div> <div> <div class="w-16 h-16 sm:w-[100px] sm:h-[100px] shadow-[1px_2px_12px_0_rgba(31,45,61,0.10)] rounded-full border border-[#e0e6ed] dark:border-[#1b2e4b] flex justify-center flex-col"> <h1 class="text-primary sm:text-3xl text-xl text-center" x-text="demo2.hours"></h1> </div> <h4 class="text-[#3b3f5c] text-[15px] mt-4 text-center dark:text-white-dark font-semibold">Hours</h4> </div> <div class="mt-5 md:mt-0"> <div class="w-16 h-16 sm:w-[100px] sm:h-[100px] shadow-[1px_2px_12px_0_rgba(31,45,61,0.10)] rounded-full border border-[#e0e6ed] dark:border-[#1b2e4b] flex justify-center flex-col"> <h1 class="text-primary sm:text-3xl text-xl text-center" x-text="demo2.minutes"></h1> </div> <h4 class="text-[#3b3f5c] text-[15px] mt-4 text-center dark:text-white-dark font-semibold">Mins</h4> </div> <div class="mt-5 md:mt-0"> <div class="w-16 h-16 sm:w-[100px] sm:h-[100px] shadow-[1px_2px_12px_0_rgba(31,45,61,0.10)] rounded-full border border-[#e0e6ed] dark:border-[#1b2e4b] flex justify-center flex-col"> <h1 class="text-primary sm:text-3xl text-xl text-center" x-text="demo2.seconds"></h1> </div> <h4 class="text-[#3b3f5c] text-[15px] mt-4 text-center dark:text-white-dark font-semibold">Sec</h4> </div> </div> </div> <!-- script --> <script> document.addEventListener("alpine:init", () => { Alpine.data("countdown", () => ({ timer2: null, demo2: { days: null, hours: null, minutes: null, seconds: null, }, setTimerDemo2() { let date = new Date(); date.setFullYear(date.getFullYear() + 1); let countDownDate = date.getTime(); this.timer2 = setInterval(() => { let now = new Date().getTime(); let distance = countDownDate - now; this.demo2.days = Math.floor(distance / (1000 * 60 * 60 * 24)); this.demo2.hours = Math.floor((distance % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)); this.demo2.minutes = Math.floor((distance % (1000 * 60 * 60)) / (1000 * 60)); this.demo2.seconds = Math.floor((distance % (1000 * 60)) / 1000); if (distance < 0) { clearInterval(this.timer2); } }, 500); }, }); }); </script>
©
. Vristo All rights reserved.